CoolEdit - Powerfull syntax coloring text editor
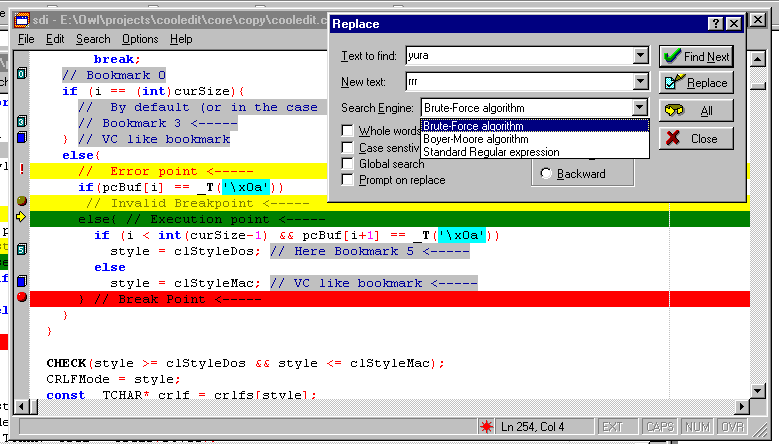
Features:
- Not limited size of file.
- Support for DOS/UNIX/MAC style of files
- Syntax coloring, support for 21 language, easy adding new languages.
- Support OLE Drag-and-Drop.
- Support Stream/Column/Line selection mode
- Has Unlimited UNDO (By default its limited by 100 operations, you can increase this
value)
- Has several built-in search engines: Standard(Brute-Force), Boyer-Moore, Regular
expression. You can easy add more.
- Option: View whitespace.
- Option: View line numbers.
- Option: Hide curet.
- Option: Hide margin.
- Option: View Inactive Selection
- Option: Look word under cursor for Find.Replace operations.
- Option: AutoIntend.
- Easy customizable.
Todo:
- Redo handling
- Fix Column selection bugs
- Fix No SmartCursor bug
- Add Code completion,Code parameters, Tooltip Symbol,
- Add Brace Matching (language depended).
- Add Smart AutoIntend (language depended).
Cool Edit implements following classes: (only importand ones)
1 |
TEditPos |
Represented text position. |
2 |
TEditRange |
Represented text range (Selection for example) |
3 |
TCoolTextBuffer |
Data provider, Holds all text lines |
4 |
TCoolScroller |
Scroller for CoolEdit |
5 |
TCoolTextWnd |
Base class, capable show text, only. |
6 |
TSyntaxParser |
Base class for syntax support |
7 |
TCoolSearchEngine |
Base class for Search support - Actual Search Engine |
8 |
TCoolEngineDescr |
Base class for Search support - Search Engine Descriptor |
9 |
TCoolFindDlg |
CoolEdit find dialog |
10 |
TCoolReplaceDlg |
CoolEdit replace dialog |
11 |
TCoolSearchWnd |
Class with search capabilities |
12 |
TCoolEdit |
Class with edit capabilities |
13 |
TCoolEditFile |
Class capable save/load files |
14 |
TCoolSearchView |
View with search capabilities |
15 |
TCoolEditView |
View with edit capabilities |
16 |
TMemComboBox |
Memory combobos |
17 |
TDynamicTextGadget |
Text gadget, for show line/column number |
18 |
TDynamicBitmapGadget |
Bitmap gadget, to show Dirty status |
19 |
TCoolDocument |
Document class |
20 |
TDragDropProxy |
Drag-and-Drop support |
21 |
TDragDropSupport |
Drag-and-Drop support |
|
|
|
TCoolTextBuffer:
Public functions:
TCoolTextBuffer(TCrLfStyle style = clStyleDos);
~TCoolTextBuffer();
bool Load(LPCTSTR filename, TCrLfStyle style = clStyleAutomatic);
bool Save(LPCTSTR filename, TCrLfStyle style = clStyleAutomatic, bool clearDirty = true);
bool Load(TFile& file, TCrLfStyle style = clStyleAutomatic);
bool Save(TFile& file, TCrLfStyle style = clStyleAutomatic, bool clearDirty = true);
void Clear();
// 'Dirty' flag
void SetDirty(bool dirty = true);
bool IsDirty() const;
// Text access functions
int GetLineCount() const;
int GetLineLength(int index) const;
LPTSTR GetLineText(int index);
TLineFlags GetLineFlags(int index) const;
int GetLineWithFlag(uint32 flag);
void SetLineFlag(int nLine, uint32 dwFlag, bool bSet, bool bRemoveFromPreviousLine =
true);
void GetText(const TEditPos& startPos, const TEditPos& endPos, LPTSTR buffer, int
buflen, LPCTSTR pszCRLF = NULL);
// Attributes
TCrLfStyle GetCRLFMode() const;
void SetCRLFMode(TCrLfStyle style);
bool IsReadOnly() const;
void EnableReadOnly(bool enable = true);
// Undo/Redo
TUndoNode* GetTopUndoNode();
TRedoNode* GetTopRedoNode();
bool CanUndo() const;
bool CanRedo() const;
bool Undo(TEditPos* newPos=0);
bool Redo(TEditPos* newPos=0);
bool AddUndoNode(TUndoNode* node);
bool AddRedoNode(TRedoNode* node);
void ClearUndoBuffer();
void ClearRedoBuffer();
bool GetUndoDescription(_TCHAR* buffer, int len, TModule* module = gModule);
bool GetRedoDescription(_TCHAR* buffer, int len, TModule* module = gModule);
// More bookmarks
int FindNextBookmarkLine(int nCurrentLine = 0);
int FindPrevBookmarkLine(int nCurrentLine = 0);
// simple search
TEditPos Search(const TEditRange& searchRange, LPCTSTR text,bool caseSens=false, bool
wholeWord=false,bool up = false);
bool DeleteText(const TEditRange& range);
bool InsertText(const TEditPos& startPos, LPCTSTR text, TEditPos& endPos);
bool AppendText(int line, LPCTSTR text, int len = -1);
void InsertLine(LPCTSTR text, int len = -1, int pos = -1);
// save and restore all format and font information
void SaveSyntaxDescr(TConfigFile& file);
void RestoreSyntaxDescr(TConfigFile& file);
void GetFont(LOGFONT& lf) const;
void SetFont(const LOGFONT& lf);
void SetUndoSize(uint newmax);
uint GetUndoSize();
uint GetUndoCnt();
void SetRedoSize(uint newmax);
uint GetRedoSize();
uint GetRedoCnt();
Imortant functions of TCoolTextWnd:
TCoolTextWnd(TWindow* parent,int id,LPCTSTR title,int x, int y,int w, int h, TModule*
module = 0);
// properties
bool IsTabsVisible() const;
void EnableTabs(bool viewTabs);
bool IsStreamSelMode() const;
bool IsLineSelMode() const;
bool IsColumnSelMode() const;
void SetSelMode(TSelType newtyp);
bool IsShowInactiveSel() const;
void EnableShowInactiveSel(bool enable = true);
bool IsItalic(int index) const;
bool IsBold(int index) const;
bool IsUnderline(int index) const;
bool IsSelectionMargin() const;
void EnableSelectionMargin(bool bSelMargin);
bool IsCaretEnable() const;
void EnableCaret(bool enable = true);
bool IsSmartCursor() const;
void EnableSmartCursor(bool enable = true);
TEditPos GetCursorPos();
bool IsSelection() const;
void EnableDragDrop(bool enable = true);
bool IsDragDrop() const;
void EnableAutoIndent(bool enable = true);
bool IsAutoIndent() const;
void ShowLineNumbers(bool enable = true);
bool IsLineNumbers() const;
int GetNumLines() const;
bool GetLine(LPTSTR str, int strSize, int lineNumber) const;
void GetFont(LOGFONT& lf) const;
void SetFont(const LOGFONT &lf);
int GetTabSize() const;
void SetTabSize(int tabSize);
TColor GetTxColor(int index) const;
TColor GetBkColor(int index) const;
TFont* GetFont(int index);
int GetLineHeight() const;
int GetLineLength(int index) const;
int GetCharWidth() const;
int GetMarginWidth() const;
void SetMarginWidth(int width);
TCoolTextBuffer::TSyntaxDescr& GetSyntaxDescr(int index) const;
TSyntaxParser* GetSyntaxParser();
void SetSyntaxParser(TSyntaxParser* parser);
void SetSelection(const TEditRange& range);
bool GetSelection(TEditRange& range);
void WordUnderCursor(LPTSTR text, uint size);
// operations
void GoToLine(int lineIndex, bool relative);
Important functions of TCoolSearchWnd:
TCoolSearchWnd(TWindow* parent,int id,LPCTSTR title,int x, int y, int w, int h,
TModule* module = 0);
virtual void SetupWindow();
virtual void DoSearch();
virtual bool Search(const TEditPos& startPos, LPCTSTR text, TFindFlags flags =
ffNone);
TCoolFindDlg::TData& GetSearchData();
TCoolFindDlg* GetSearchDialog();
void SetSearchDialog(TCoolFindDlg* searchdialog);
uint GetSearchCmd();
void SetSearchCmd(uint searchcmd);
Imortant functions of TCoolEdit:
TCoolEdit(TWindow* parent,int id,LPCTSTR title,int x, int y, int w, int h, TModule*
module = 0);
Imortant functions of TCoolEditFile:
LPCTSTR GetFileName() const;
void SetFileName(LPCTSTR fileName);
TOpenSaveDialog::TData& GetFileData();
void SetFileData(const TOpenSaveDialog::TData& fd);
virtual void FileNew();
virtual bool FileOpen(LPCTSTR filename);
virtual bool FileSave();
virtual bool FileSaveAs();
Revised: August 06, 2001.